Bash 数组
Bash 数组
在本主题中,我们将演示 bash 数组的基础知识以及它们如何在 bash shell 脚本中使用。
数组可以是定义为相似类型元素的集合。与大多数编程语言不同,bash 脚本中的数组不必是相似元素的集合。由于 Bash 不区分字符串和数字,因此数组可能同时包含字符串和数字。
Bash 不提供对多维数组的支持;我们不能拥有本身就是数组的元素。 Bash 支持一维数字索引数组以及关联数组。要访问最后一个数字索引的数组,我们可以使用负索引。 '-1' 的索引将被视为最后一个元素的引用。我们可以在一个数组中使用多个元素。
Bash 数组声明
Bash 中的数组可以通过以下方式声明:
创建数字索引数组
我们可以将任何变量用作索引数组而无需声明。
显式声明变量为 Bash数组,使用关键字'declare',语法可以定义为:
where,
ARRAY_NAME 表示我们将分配给数组的名称。
注意: 在 Bash 中命名变量的规则与命名数组相同。
创建索引数组的一般方法可以定义为以下形式:
ARRAY_NAME[index_1]=value_1
ARRAY_NAME[index_2]=value_2
ARRAY_NAME[index_n]=value_n
其中关键字"index"用于定义正整数。
创建关联数组
与数字索引数组不同,首先声明关联数组。我们可以使用关键字"declare"和-A(大写)选项来声明关联数组。语法可以定义为:
创建关联数组的一般方法可以定义为以下形式:
declare-A ARRAY_NAME
ARRAY_NAME[index_foo]=value_foo
ARRAY_NAME[index_bar]=value_bar
ARRAY_NAME[index_xyz]=value_xyz
其中index_用于定义任意字符串。
我们也可以这样写上面的形式:
declare-A ARRAY_NAME
ARRAY_NAME=(
[index_foo]=value_foo
[index_bar]=value_bar
[index_xyz]=value_xyz
)
Bash 数组初始化
要初始化 Bash 数组,我们可以使用赋值运算符(=),通过指定括号内的元素列表,用空格分隔,如下所示:
ARRAY_NAME=(element_1st element_2nd element_Nth)
注意: 此处,第一个元素的索引为 0。此外,赋值运算符(=) 周围不应有空格。
访问 Bash 数组的元素
要访问 Bash 数组的元素,我们可以使用以下语法:
打印 Bash 数组
我们可以使用关键字 'declare' 和一个 '-p' 选项来打印包含所有索引和详细信息的 Bash 数组的所有元素。打印 Bash Array 的语法可以定义为:
数组操作
一旦分配了数组,我们就可以对其执行一些有用的操作。我们可以显示它的键和值,也可以通过添加或删除元素来修改它:
引用元素
要引用单个元素,我们需要知道元素的索引号。我们可以使用以下语法引用或打印任何元素:
注意: 需要大括号 ${} 以避免 shell 的文件名扩展操作符。
例如,让我们打印一个索引为 2 的数组元素:
Bash Script
#!/bin/bash
#Script to print an element of an array with an index of 2
#declaring the array
declare-a example_array=( "Welcome""To""lidihuo" )
#printing the element with index of 2
echo ${example_array[2]}
输出
如果我们在指定的索引处使用@或*,它将扩展到数组的所有成员。要打印所有元素,我们可以使用以下形式:
Bash Script
#!/bin/bash
#Script to print all the elements of the array
#declaring the array
declare-a example_array=( "Welcome""To""lidihuo" )
#Printing all the elements
echo "${example_array[@]}"
输出
使用@和*的唯一区别是使用@时表格用双引号括起来。在第一种情况下(使用 @ 时),扩展为数组的每个元素提供一个单词的结果。在"for循环"的帮助下可以更好地描述它。假设我们有一个包含三个元素的数组,"Welcome"、"To"和"lidihuo":
$ example_array= (Welcome to lidihuo)
使用@ 应用循环:
for i in "${example_array[@]}"; do echo "$i"; done
它会产生以下结果:
应用带有 * 的循环,将产生一个结果,将数组的所有元素保存为一个单词:
理解@和*的用法很重要,因为它在使用表单迭代数组元素时很有用。
打印数组的键
我们还可以检索和打印索引或关联数组中使用的键,而不是它们各自的值。可以通过添加 !数组名前的运算符如下:
示例
#!/bin/bash
#Script to print the keys of the array
#Declaring the Array
declare-a example_array=( "Welcome""To""lidihuo" )
#Printing the Keys
echo "${!example_array[@]}"
输出
求数组长度
我们可以用下面的形式统计数组中包含的元素个数:
示例
#!/bin/bash
#Declaring the Array
declare-a example_array=( "Welcome""To""lidihuo" )
#Printing Array Length
echo "The array contains ${#example_array[@]} elements"
输出
The array contains 3 elements
循环遍历数组
遍历数组中每一项的一般方法是使用"for循环"。
示例
#!/bin/bash
#Script to print all keys and values using loop through the array
declare-a example_array=( "Welcome""To""lidihuo" )
#Array Loop
for i in "${!example_array[@]}"
do
echo The key value of element "${example_array[$i]}" is "$i"
done
输出
另一种常用的循环方法数组是检索数组的长度并使用C风格的循环:
Bash Script
#!/bin/bash
#Script to loop through an array in C-style
declare-a example_array=( "Welcome""To""lidihuo" )
#Length of the Array
length=${#example_array[@]}
#Array Loop
for (( i=0; i < ${length}; i++ ))
do
echo $i ${example_array[$i]}
done
输出
添加数组元素
我们可以选择通过分别指定元素的索引或关联键,将元素添加到索引或关联数组。要在 bash 中将新元素添加到数组中,我们可以使用以下形式:
ARRAY_NAME[index_n]="New Element"
示例
#!/bin/bash
#Declaring an array
declare-a example_array=( "Java""Python""PHP""HTML" )
#Adding new element
example_array[4]="JavaScript"
#Printing all the elements
echo "${example_array[@]}"
输出
Java Python PHP HTML JavaScript
另一种向数组添加新元素的方法是使用 += 运算符。此方法中无需指定索引。我们可以通过以下方式在数组中添加一个或多个元素:
示例
#!/bin/bash
#Declaring the Array
declare-a example_array=( "Java""Python""PHP" )
#Adding new elements
example_array+=( JavaScript CSS SQL )
#Printing all the elements
echo "${example_array[@]}"
输出
Java Python PHP JavaScript CSS SQL
更新数组元素
我们可以通过将新值分配给现有数组的索引值来更新数组元素。让我们用元素"lidihuo"更改索引 4 处的数组元素。
示例
#!/bin/bash
#Script to update array element
#Declaring the array
declare-a example_array=( "We""welcome""you""on""SSSIT" )
#Updating the Array Element
example_array[4]=lidihuo
#Printig all the elements of the Array
echo ${example_array[@]}
输出
We welcome you on lidihuo
从数组中删除一个元素
如果我们想从数组中删除一个元素,我们必须知道它的索引或键(如果是关联数组)。可以使用"unset"命令删除元素:
下面显示了一个示例,让您更好地理解这个概念:
示例
#!/bin/bash
#Script to delete the element from the array
#Declaring the array
declare-a example_array=( "Java""Python""HTML""CSS""JavaScript" )
#Removing the element
unset example_array[1]
#Printing all the elements after deletion
echo "${example_array[@]}"
输出
在这里,我们创建了一个由五个元素组成的简单数组,"Java"、"Python"、"HTML"、"CSS"和"JavaScript"。然后我们通过使用"unset"并引用它的索引从数组中删除元素"Python"。元素"Python"的索引是'1',因为bash数组从0开始。如果我们在删除元素后检查数组的索引,我们可以看到被删除元素的索引丢失了。我们可以通过在脚本中添加以下命令来检查索引:
echo ${!example_array[@]}
输出将如下所示:
这个概念也适用于关联数组。
删除整个数组
删除整个数组是一项非常简单的任务。它可以通过将数组名称作为参数传递给 'unset' 命令来执行,而无需指定索引或键。
示例
#!/bin/bash
#Script to delete the entire Array
#Declaring the Array
declare-a example_array=( "Java""Python""HTML""CSS""JavaScript" )
#Deleting Entire Array
unset example_array
#Printing the Array Elements
echo ${!example_array[@]}
#Printing the keys
echo ${!example_array[@]}
输出
如果没有输出我们尝试打印上述脚本的内容。返回空结果,因为数组不再存在。
切片数组元素
Bash 数组也可以从给定的起始索引到结尾切片
要将数组从起始索引 'm' 切片到结束索引 'n',我们可以使用以下语法:
SLICED_ARRAY=(${ARRAY_NAME[@]:m:n}")
示例
#!/bin/bash
#Script to slice Array Element from index 1 to index 3
#Declaring the Array
example_array=( "Java""Python""HTML""CSS""JavaScript" )
#Slicing the Array
sliced_array=("${example_array[@]:1:3}")
#Applying for loop to iterate over each element in Array
for i in "${sliced_array[@]}"
do
echo $i
done
输出
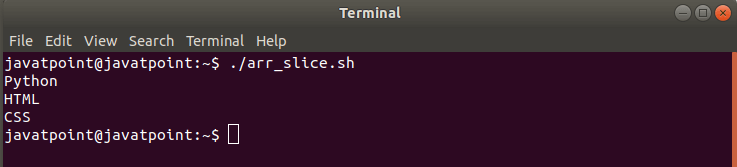