对称和不对称密码
对称和不对称密码详细操作教程
在本章中,让我们详细讨论对称和非对称密码。
对称密码学
在这种类型中,加密和解密过程使用相同的密钥。它也称为
秘密密钥密码学。对称密码学的主要特征如下-
它更简单,更快。
双方以安全的方式交换密钥。
缺点
对称加密的主要缺点是,如果密钥泄露给入侵者,则可以轻松更改消息,这被认为是危险因素。
数据加密标准(DES)
最受欢迎的对称密钥算法是数据加密标准(Data Encryption Standard,DES),Python包含一个包含DES算法背后逻辑的软件包。
安装
在Python中安装DES软件包
pyDES 的命令是-
# Filename : example.py
# Copyright : 2020 By Lidihuo
# Author by : www.lidihuo.com
# Date : 2020-08-28
pip install pyDES
DES算法的简单程序实现如下-
# Filename : example.py
# Copyright : 2020 By Lidihuo
# Author by : www.lidihuo.com
# Date : 2020-08-28
import pyDes
data = "DES Algorithm Implementation"
k = pyDes.des("DESCRYPT", pyDes.CBC, "\0\0\0\0\0\0\0\0", pad=None, padmode=pyDes.PAD_PKCS5)
d = k.encrypt(data)
print "Encrypted: %r" % d
print "Decrypted: %r" % k.decrypt(d)
assert k.decrypt(d) == data
它需要变量
padmode ,该变量按照DES算法的实现获取所有软件包,并以指定的方式进行加密和解密。
输出
由于上面给出的代码,您可以看到以下输出-
非对称密码学
它也称为
公钥密码学。它以对称密码学的相反方式工作。这意味着它需要两个密钥:一个用于加密,另一个用于解密。公钥用于加密,私钥用于解密。
缺点
由于其密钥长度,它有助于降低加密速度。
密钥管理至关重要。
以下Python中的程序代码说明了使用RSA算法的非对称密码术的工作及其实现-
# Filename : example.py
# Copyright : 2020 By Lidihuo
# Author by : www.lidihuo.com
# Date : 2020-08-28
from Crypto import Random
from Crypto.PublicKey import RSA
import base64
def generate_keys():
# key length must be a multiple of 256 and >= 1024
modulus_length = 256*4
privatekey = RSA.generate(modulus_length, Random.new().read)
publickey = privatekey.publickey()
return privatekey, publickey
def encrypt_message(a_message , publickey):
encrypted_msg = publickey.encrypt(a_message, 32)[0]
encoded_encrypted_msg = base64.b64encode(encrypted_msg)
return encoded_encrypted_msg
def decrypt_message(encoded_encrypted_msg, privatekey):
decoded_encrypted_msg = base64.b64decode(encoded_encrypted_msg)
decoded_decrypted_msg = privatekey.decrypt(decoded_encrypted_msg)
return decoded_decrypted_msg
a_message = "This is the illustration of RSA algorithm of asymmetric cryptography"
privatekey , publickey = generate_keys()
encrypted_msg = encrypt_message(a_message , publickey)
decrypted_msg = decrypt_message(encrypted_msg, privatekey)
print "%s - (%d)" % (privatekey.exportKey() , len(privatekey.exportKey()))
print "%s - (%d)" % (publickey.exportKey() , len(publickey.exportKey()))
print " Original content: %s - (%d)" % (a_message, len(a_message))
print "Encrypted message: %s - (%d)" % (encrypted_msg, len(encrypted_msg))
print "Decrypted message: %s - (%d)" % (decrypted_msg, len(decrypted_msg))
输出
执行上面给出的代码时,您可以找到以下输出-
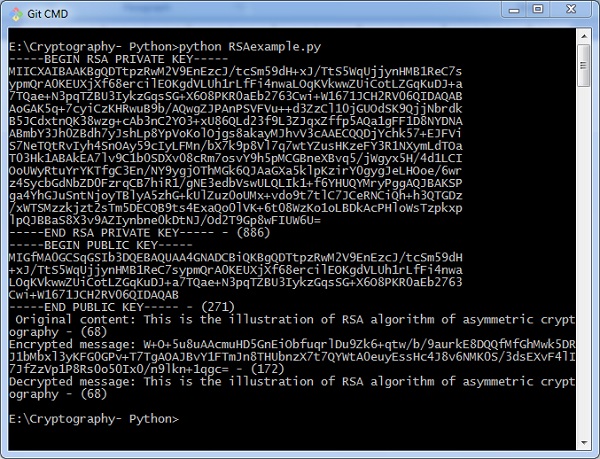