Angular8 动画
动画为 Web 应用程序提供了令人耳目一新的外观和丰富的用户交互。在 HTML 中,动画基本上是在特定时间段内将 HTML 元素从一种 CSS 样式转换为另一种样式。例如,可以通过更改其宽度和高度来放大图像元素。
如果图像的宽度和高度在一段时间内逐步从初始值更改为最终值,例如 10 秒,那么我们将获得动画效果。因此,动画的范围取决于 CSS 提供的功能/属性来设置 HTML 元素的样式。
Angular 提供了一个单独的模块
BrowserAnimationModule 来执行动画。
BrowserAnimationModule 提供了一种简单明了的动画制作方法。
配置动画模块
让我们在本章中学习如何配置动画模块。
按照下面提到的步骤在应用程序中配置动画模块
BrowserAnimationModule。
在 AppModule 中导入
BrowserAnimationModule。
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
@NgModule({
imports: [
BrowserModule,
BrowserAnimationsModule
],
declarations: [ ],
bootstrap: [ ]
})
export class AppModule { }
在相关组件中导入动画功能。
import { state, style, transition, animate, trigger } from '@angular/animations'
在相关组件中添加
animations 元数据属性。
@Component({
animations: [
// animation functionality goes here
]
})
export class MyAnimationComponent
概念
在angular中,我们需要了解五个核心概念以及它们之间的关系来做动画。
状态
State 指的是组件的具体状态。一个组件可以有多个定义的状态。状态是使用 state() 方法创建的。 state() 方法有两个参数。
name-状态的唯一名称。
style-使用 style() 方法定义的状态样式。
animations: [
...
state('start', style( { width: 200px; } ))
...
]
这里,
start 是状态的名称。
风格
Style 是指在特定状态下应用的 CSS 样式。 style() 方法用于设置组件特定状态的样式。它使用 CSS 属性并且可以有多个项目。
animations: [
...
state('start', style( { width: 200px; opacity: 1 } ))
...
]
这里,
start 状态定义了两个 CSS 属性,
width 值为 200px,opacity 值为 1、
过渡
Transition 指的是从一种状态到另一种状态的转换。动画可以有多个过渡。每个转换都是使用 transition() 函数定义的。 transition() 有两个参数。
指定两个过渡状态之间的方向。例如,start => end 表示初始状态为start,最终状态为end。实际上,它是一个功能丰富的表达式。
使用 animate() 函数指定动画细节。
animations: [
...
transition('start => end', [
animate('1s')
])
...
]
这里,
transition() 函数定义了从开始状态到结束状态的转换,动画在
animate() 方法中定义。
动画
动画定义了从一种状态到另一种状态的转换方式。
animation() 函数用于设置动画细节。
animate() 采用以下表达式形式的单个参数-
duration-指转换的持续时间。表示为1s、100ms等,
delay-指开始转换的延迟时间。它的表达方式类似于 duration
easing-指的是如何在给定的持续时间内加速/减速过渡。
触发
每个动画都需要一个触发器来启动动画。 trigger() 方法用于将状态、样式、过渡和动画等所有动画信息设置在一处,并为其赋予唯一的名称。唯一名称将进一步用于触发动画。
animations: [
trigger('enlarge', [
state('start', style({
height: '200px',
})),
state('end', style({
height: '500px',
})),
transition('start => end', [
animate('1s')
]),
transition('end => start', [
animate('0.5s')
]) ]),
]
这里,
放大是给特定动画的唯一名称。它有两种状态和相关的样式。它有两个过渡,一个从开始到结束,另一个从结束到开始。 End to start 状态做相反的动画。
触发器 可以附加到如下指定的元素-
<div [@triggerName]="expression">...</div>;
例如
<img [@enlarge]="isEnlarge ? 'end' : 'start'">...</img>;
这里,
@enlarge-触发器设置为图像标记并附加到表达式。
如果 isEnlarge 值更改为 true,则将设置 end 状态并触发 start => 结束转换。
如果 isEnlarge 值更改为 false,则将设置 start 状态并触发 end => start 转换。
简单动画示例
让我们编写一个新的 angular 应用程序,通过放大具有动画效果的图像来更好地理解动画概念。
打开命令提示符并创建新的 Angular 应用程序。
cd /go/to/workspace
ng new animation-app
cd animation-app
在
AppModule (src/app/app.module.ts)
中配置
BrowserAnimationModule
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core'
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
import { AppComponent } from './app.component'; @NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
BrowserAnimationsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
打开
AppComponent (src/app/app.component.ts) 并导入必要的动画功能。
import { state, style, transition, animate, trigger } from '@angular/animations';
添加动画功能,在放大/缩小图像时为图像添加动画。
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
animations: [
trigger('enlarge', [
state('start', style({
height: '150px'
})),
state('end', style({
height: '250px'
})),
transition('start => end', [
animate('1s 2s')
]),
transition('end => start', [
animate('1s 2s')
])
])
]
})
打开
AppComponent 模板,
src/app/app.component.html 并删除示例代码。然后,添加一个包含应用程序标题、图像和按钮以放大/缩小图像的标题。
<h1>{{ title }}</h1>
<img src="assets/puppy.jpeg" style="height: 200px" /> <br />
<button>{{ this.buttonText }}</button>
编写一个函数来改变动画表达。
export class AppComponent {
title = 'Animation Application';
isEnlarge: boolean = false;
buttonText: string = "Enlarge";
triggerAnimation() {
this.isEnlarge = !this.isEnlarge;
if(this.isEnlarge)
this.buttonText = "Shrink";
else
this.buttonText = "Enlarge";
}
}
在图像标签中附加动画。另外,附加按钮的点击事件。
<h1>{{ title }}</h1>
<img [@enlarge]="isEnlarge ? 'end' : 'start'" src="assets/puppy.jpeg" style="height: 200px" />
<br />
<button (click)='triggerAnimation()'>{{ this.buttonText }}</button>
完整的
AppComponent代码如下-
import { Component } from '@angular/core';
import { state, style, transition, animate, trigger } from '@angular/animations';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
animations: [
trigger('enlarge', [
state('start', style({
height: '150px'
})),
state('end', style({
height: '250px'
})),
transition('start => end', [
animate('1s 2s')
]),
transition('end => start', [
animate('1s 2s')
])
])
]
})
export class AppComponent {
title = 'Animation Application';
isEnlarge: boolean = false;
buttonText: string = "Enlarge";
triggerAnimation() {
this.isEnlarge = !this.isEnlarge;
if(this.isEnlarge)
this.buttonText = "Shrink";
else
this.buttonText = "Enlarge";
}
}
完整的 AppComponent 模板代码如下-
<h1>{{ title }}</h1>
<img [@enlarge]="isEnlarge ? 'end' : 'start'" src="assets/puppy.jpeg" style="height: 200px" />
<br />
<button (click)='triggerAnimation()'>{{ this.buttonText }}</button>
使用以下命令运行应用程序-
点击放大按钮,它会放大带有动画的图像。结果如下图所示-
再次单击按钮将其缩小。结果如下图所示-
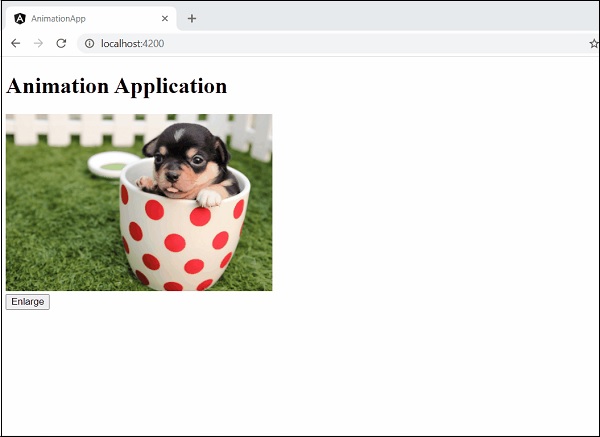