TestNG 注释属性
TestNG注解属性
在TestNG中编写测试用例时,需要在测试方法之前提及@Test注解。
@Test
public void testcase1()
{
System.out.println("this is testcase1");
}
在上面的代码中,我们在测试方法之前指定了@Test注解,即testcase1()。
我们也可以在@Test中显式指定属性强>注释。测试属性是测试特定的,它们在 @Test 注释旁边的右侧指定。
@Test(attribute="value")
public void testcase2()
{
System.out.println("this is testcase2");
}
一些常见的属性描述如下:
description
timeOut
priority
dependsOnMethods
enabled
groups
description
这是一个字符串,附加在@Test注解上,描述有关测试的信息。
让我们通过一个例子来理解。
package com.lidihuo;
import org.testng.annotations.Test;
public class Class1
{
@Test(description="this is testcase1")
public void testcase1()
{
System.out.println("HR");
}
@Test(description="this is testcase2")
public void testcase2()
{
System.out.println("Software Developer");
}
@Test(description="this is testcase3")
public void testcase3()
{
System.out.println("QA Analyst");
}
}
在上面的代码中,我们在每个测试中都添加了描述属性。 "描述"属性提供有关测试的信息。
dependsOnMethods
当第二个测试方法想要依赖第一个时测试方法,那么这可以通过使用"dependOnMethods"属性来实现。如果第一个测试方法失败,那么第一个测试方法的依赖方法,即第二个测试方法将不会运行。
我们通过一个例子来理解。
第一种情况: 在参数中传递单个值时。
package com.lidihuo;
import org.testng.annotations.Test;
public class Class1
{
@Test
public void WebStudentLogin()
{
System.out.println("Student login through web");
}
@Test
public void MobileStudentLogin()
{
System.out.println("Student login through mobile");
}
@Test(dependsOnMethods= {"WebStudentLogin"})
public void APIStudentLogin()
{
System.out.println("Student login through API");
}
}
我们知道TestNG是按字母顺序执行测试方法的,所以在上面的程序中,APIStudentLogin()会先执行。但是,我们希望在 APIStudentLogin() 方法执行之前执行 WebStudentLogin() 方法,因此这只能通过"dependsOnMethods"属性来实现。在上面的程序中,我们在 APIStudentLogin() 测试方法中指定了"dependsOnMethods"属性,其值为"WebStudentLogin",这意味着 WebStudentLogin() 方法将在 APIStudentLogin() 方法之前执行。
输出
在上面的输出中,MobileStudentLogin( ) 在 WebStudentLogin() 方法之前运行,因为 TestNG 按字母顺序运行测试方法。
第二种情况: 当在参数中传递多个值时。
package com.lidihuo;
import org.testng.annotations.Test;
public class Depends_On_Groups
{
@Test(dependsOnMethods= {"testcase3","testcase2"})
public void testcase1()
{
System.out.println("this is test case1");
}
@Test
public void testcase2()
{
System.out.println("this is test case2");
}
@Test
public void testcase3()
{
System.out.println("this is test case3");
}
}
上面代码中,testcase1()依赖于两个方法,即testcase2()和testcase3(),也就是说这两个方法会在testcase1()之前执行。
输出
priority
当未指定"优先级"属性时,TestNG 将按字母顺序运行测试用例。优先级决定了测试用例的执行顺序。优先级可以保存-5000到5000之间的整数值。当设置了优先级时,优先级最低的测试用例将首先运行,优先级最高的测试用例将最后执行。假设我们有三个测试用例,它们的优先级值为-5000、0、15,那么执行的顺序将是0、15、5000。如果不指定优先级,则默认优先级为0。
我们通过一个例子来理解。
package com.lidihuo;
import org.testng.annotations.Test;
public class Fruits
{
@Test
public void mango()
{
System.out.println("I am Mango");
}
@Test(priority=2)
public void apple()
{
System.out.println("I am Apple");
}
@Test(priority=1)
public void watermelon()
{
System.out.println("I am Watermelon");
}
}
在上面的代码中,mango()测试方法的默认优先级是0,所以会先执行。 watermelon() 测试方法会在 mango() 方法之后运行,因为 watermelon() 测试方法的优先级为 2、 apple() 测试方法的优先级最高,所以它会最后执行。
输出
enabled
"enabled"属性包含布尔值。默认情况下,其值为 true。如果你想跳过一些测试方法,那么你需要明确指定'false'值。
让我们通过一个例子来理解。
package com.lidihuo;
import org.testng.annotations.Test;
public class Programming_languages
{
@Test
public void c_language()
{
System.out.println("C language");
}
@Test(enabled=false)
public void jira()
{
System.out.println("JIRA is a testing tool");
}
@Test
public void java()
{
System.out.println("JAVA language");
}
}
上面代码中,jira()测试方法中的enabled属性值为false,因此不会调用该方法。
输出
groups
'groups' 属性用于对属于同一功能的不同测试用例进行分组。
让我们通过一个例子来理解。
package com.lidihuo;
import org.testng.annotations.Test;
public class Software_Company
{
@Test(groups= {"software company"})
public void infosys()
{
System.out.println("Infosys");
}
@Test
public void technip()
{
System.out.println("Technip India Ltd");
}
@Test(groups= {"software company"})
public void wipro()
{
System.out.println("Wipro");
}
}
testng.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd">
<suite name="test_suite">
<test name="Software Company">
<groups>
<run>
<include name="software company"/>
</run>
</groups>
<classes>
<class name="com.lidihuo.Software_Company"/>
</classes>
</test> <!--Test-->
</suite> <!--Suite-->
输出
timeOut
如果其中一个测试用例因其他测试用例失败而花费很长时间。为了克服这种情况,您需要将测试用例标记为失败以避免其他测试用例的失败。超时时间是提供给测试用例以完全执行其测试用例的时间段。
让我们通过一个例子来理解。
package com.lidihuo;
import org.testng.annotations.Test;
public class Timeout_program
{
@Test(timeOut=200)
public void testcase1() throws InterruptedException
{
Thread.sleep(500);
System.out.println("this is testcase1");
}
@Test
public void testcaes2()
{
System.out.println("this is testcase2");
}
@Test
public void testcase3()
{
System.out.println("this is testcase3");
}
}
在上面的代码中,在 testcase1() 方法中,我们有 Thread.sleep(500) 这意味着 testcase1() 方法将在 500 毫秒后执行,但我们提供了值为 200 的 timeOUT 属性testcase1() 将在 200 毫秒后失败。
testng.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd">
<suite name="test_suite">
<test name="TimeOut Program">
<classes>
<class name="com.lidihuo.Timeout_program"/>
</classes>
</test> <!--Test-->
</suite> <!--Suite-->
输出
上图显示一个测试用例失败,其他测试用例通过。
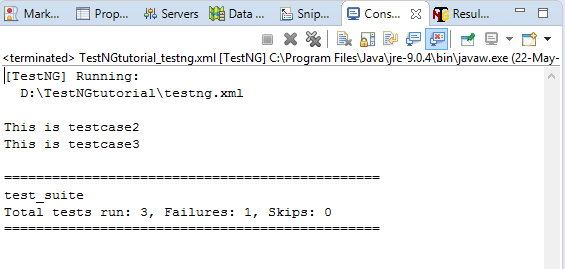