比较两个字符串
C程序比较两个字符串
可以使用字符串函数或不使用字符串函数来比较字符串。首先,我们将研究如何在字符串函数(即 strcmp())中比较字符串,该函数在 string.h 头文件中定义。
使用字符串函数进行字符串比较
在 string.h 头文件中预定义的字符串函数是 strcmp()函数。 strcmp()函数将两个字符串作为参数,此函数返回一个整数值,该整数值可以为零,正或负 >。
strcmp()函数的语法如下:
int strcmp (const char* str1, const char* str2);
在上述语法中,两个参数作为字符串传递,即 str1 和 str2 ,返回类型为 int 表示strcmp()返回一个整数值。
strcmp()函数比较两个字符串的字符。如果两个字符串的第一个字符相同,那么将继续此比较过程,直到比较所有字符或指针指向空字符'\ 0'。
可能的返回值来自strcmp()函数
返回值 |
说明 |
0 |
两个字符串都相等时。 |
< 0 |
如果第一个字符串的字符的ASCII值小于第二个字符串的字符的ASCII值,则该函数将返回负值。 |
> 0 |
如果第一个字符串的字符的ASCII值大于第二个字符串的字符的ASCII值,则该函数将返回正值。 |
让我们通过一个例子来理解。
#include <stdio.h>
#include<string.h>
int main()
{
char str1[20]; // declaration of char array
char str2[20]; // declaration of char array
int value; // declaration of integer variable
printf("Enter the first string : ");
scanf("%s",str1);
printf("Enter the second string : ");
scanf("%s",str2);
// comparing both the strings using strcmp() function
value=strcmp(str1,str2);
if(value==0)
printf("strings are same");
else
printf("strings are not same");
return 0;
}
上述程序分析
我们声明了两个char类型的数组,即str1和str2、我们将用户输入作为字符串。
我们使用 strcmp()函数(即 strcmp(str1,str2))比较字符串。此函数将同时比较字符串str1和str2、如果函数返回0,则值表示两个字符串相同,否则字符串不相等。
输出:
不使用strcmp()函数进行字符串比较
#include <stdio.h>
int compare(char[],char[]);
int main()
{
char str1[20]; // declaration of char array
char str2[20]; // declaration of char array
printf("Enter the first string : ");
scanf("%s",str1);
printf("Enter the second string : ");
scanf("%s",str2);
int c= compare(str1,str2); // calling compare() function
if(c==0)
printf("strings are same");
else
printf("strings are not same");
return 0;
}
// Comparing both the strings.
int compare(char a[],char b[])
{
int flag=0,i=0; // integer variables declaration
while(a[i]!='\0' &&b[i]!='\0') // while loop
{
if(a[i]!=b[i])
{
flag=1;
break;
}
i++;
}
if(flag==0)
return 0;
else
return 1;
}
上述程序分析
在上面,我们声明了两个char类型的数组,并将用户输入作为字符串。
我们定义了一个compare()函数,该函数将用户输入的字符串作为参数,并对两个字符串进行比较。如果函数返回0,则表示两个字符串相等,否则两个字符串不相等。
输出:
使用指针比较字符串
#include <stdio.h>
int stringcompare(char*,char*);
int main()
{
char str1[20]; // declaration of char array
char str2[20]; // declaration of char array
printf("Enter the first string : ");
scanf("%s",str1);
printf("\nEnter the second string : ");
scanf("%s",str2);
int compare=stringcompare(str1,str2); // calling stringcompare() function.
if(compare==0)
printf("strings are equal");
else
printf("strings are not equal");
return 0;
}
// Comparing both the strings using pointers
int stringcompare(char *a,char *b)
{
int flag=0;
while(*a!='\0' && *b!='\0') // while loop
{
if(*a!=*b)
{
flag=1;
}
a++;
b++;
}
if(flag==0)
return 0;
else
return 1;
}
上述程序分析
我们创建了两个char类型为str1和str2的数组。我们将用户输入作为字符串。
我们定义了一个stringcompare()函数,该函数将两个char类型的指针作为参数。 " a"指针保存str1的地址," b"指针保存str2的地址。在函数内部,我们创建了一个while循环,该循环将执行直到指针a或b均未到达空字符为止。
输出:
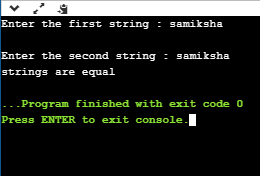