BabylonJS 视差映射
视差映射也称为偏移映射。它使用高度贴图作为材质纹理上的偏移量,以突出几何体表面的浮雕效果。在 3D 世界中,应用了深度的石墙将具有更明显的外观,并且对最终用户来说看起来更逼真。在更陡峭的视角下,纹理坐标位移更多,随着视图的变化产生视差效应导致的深度错觉。
视差贴图与标准材质一起使用。我们在标准材料章节中了解了这一点。
有 3 个属性存在于并行映射中。
material.useParallax = true;-这将启用视差映射。要使用此属性,您需要先为材质指定凹凸纹理。
material.useParallaxOcclusion = true;-要使用此属性,您必须将 useParallax 设置为 true。它启用视差遮挡。
material.parallaxScaleBias = 0.1;-将深度的缩放因子应用到网格上。0.05 和 .1 之间的值适用于视差。对于遮挡,可以达到 0.2、
演示
<!doctype html>
<html>
<head>
<meta charset = "utf-8">
<title>BabylonJs-Basic Element-Creating Scene</title>
<script src = "babylon.js"></script>
<style>
canvas {width: 100%; height: 100%;}
</style>
</head>
<body>
<canvas id = "renderCanvas"></canvas>
<script type = "text/javascript">
var canvas = document.getElementById("renderCanvas");
var engine = new BABYLON.Engine(canvas, true);
var createScene = function() {
// this creates a basic Babylon Scene object (non-mesh)
var scene = new BABYLON.Scene(engine);
// this creates and positions a free camera (non-mesh)
var camera = new BABYLON.ArcRotateCamera("camera1", 0, Math.PI / 2, 100, new BABYLON.Vector3(0, 0, 0), scene);
camera.attachControl(canvas, false);
// this targets the camera to scene origin
camera.setTarget(BABYLON.Vector3.Zero());
// this creates a light, aiming 0,1,0-to the sky (non-mesh)
var light = new BABYLON.HemisphericLight("light1", new BABYLON.Vector3(0, 1, 0), scene);
// default intensity is 1. Let's dim the light a small amount
light.intensity = 0.7;
var mesh = BABYLON.Mesh.CreateBox("box01", 25, scene);
mesh.position = new BABYLON.Vector3(0, 0, 0);
var brickWallDiffURL = "images/a1.png";
var brickWallNHURL = "images/a2.png";
var stoneDiffURL = "images/pebble.jpg";
var stoneNHURL = "images/a3.png";
var stoneDiffuseTexture = new BABYLON.Texture(stoneDiffURL, scene);
var stoneNormalsHeightTexture = new BABYLON.Texture(stoneNHURL, scene);
var wallDiffuseTexture = new BABYLON.Texture(brickWallDiffURL, scene);
var wallNormalsHeightTexture = new BABYLON.Texture(brickWallNHURL, scene);
var normalsHeightTexture = stoneNormalsHeightTexture;
var material = new BABYLON.StandardMaterial("mtl01", scene);
material.diffuseTexture = stoneDiffuseTexture;
material.bumpTexture = stoneNormalsHeightTexture;
material.useParallax = true;
material.useParallaxOcclusion = true;
material.parallaxScaleBias = 0.1;
material.specularPower = 1000.0;
material.specularColor = new BABYLON.Color3(0.5, 0.5, 0.5);
mesh.material = material;
return scene;
};
var scene = createScene();
engine.runRenderLoop(function() {
scene.render();
});
</script>
</body>
</html>
输出
上面的代码行将生成以下输出-
在这个演示中,我们使用了图像
a1.png、a2.png、pebble.jpg 和
a3.png。图片保存在本地的images/文件夹中,也贴在下面供参考。您可以下载任何您选择的图片并在演示链接中使用。
图片/a1.png
图片/a2.png
图片/pebble.jpg
图像/a3.png
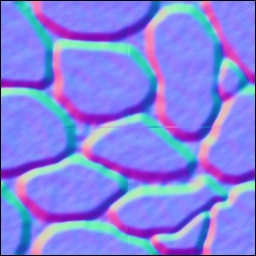