GWT UiBinder
简介
UiBinder 是一个框架,旨在分离用户界面的功能和视图。
UiBinder 框架允许开发人员将 gwt 应用程序构建为 HTML 页面,并在其中配置 GWT 小部件。
UiBinder 框架使与比 Java 源代码更熟悉 XML、HTML 和 CSS 的 UI 设计人员更容易协作
UIBinder 提供了一种定义用户界面的声明方式。
UIBinder 将程序逻辑与 UI 分开。
UIBinder 类似于 JSP 之于 Servlet。
UiBinder 工作流程
步骤 1-创建 UI 声明 XML 文件
创建基于 XML/HTML 的用户界面声明文件。我们在示例中创建了一个
Login.ui.xml 文件。
<ui:UiBinder xmlns:ui = 'urn:ui:com.google.gwt.uibinder'
xmlns:gwt = 'urn:import:com.google.gwt.user.client.ui'
xmlns:res = 'urn:with:com.tutorialspoint.client.LoginResources'>
<ui:with type = "com.tutorialspoint.client.LoginResources" field = "res">
</ui:with>
<gwt:HTMLPanel>
...
</gwt:HTMLPanel>
</ui:UiBinder>
第 2 步-使用 ui:field 进行后期绑定
在 XML/HTML 元素中使用 ui:field 属性将 XML 中的 UI 字段与 JAVA 文件中的 UI 字段相关联,以便以后绑定。
<gwt:Label ui:field = "completionLabel1" />
<gwt:Label ui:field = "completionLabel2" />
第 3 步-创建 UI XML 的 Java 副本
通过扩展复合小部件创建基于 Java 的基于 XML 的布局对应物。我们在示例中创建了一个
Login.java 文件。
package com.tutorialspoint.client;
...
public class Login extends Composite {
...
}
步骤 4-使用 UiField 注解绑定 Java UI 字段
在
Login.java 中使用 @UiField 注释来指定对应的类成员以绑定到
Login.ui.xml
中基于 XML 的字段
public class Login extends Composite {
...
@UiField
Label completionLabel1;
@UiField
Label completionLabel2;
...
}
步骤 5-使用 UiTemplate 注释将 Java UI 与 UI XML 绑定
指示 GWT 使用@UiTemplate 注释绑定基于 Java 的组件
Login.java 和基于 XML 的布局
Login.ui.xml
public class Login extends Composite {
private static LoginUiBinder uiBinder = GWT.create(LoginUiBinder.class);
/*
* @UiTemplate is not mandatory but allows multiple XML templates
* to be used for the same widget.
* default file loaded will be <class-name>.ui.xml
*/
@UiTemplate("Login.ui.xml")
interface LoginUiBinder extends UiBinder<Widget, Login> {
}
...
}
第 6 步-创建 CSS 文件
创建一个外部 CSS 文件
Login.css 和基于 Java 的资源
LoginResources.java 文件等效于 css 样式
.blackText {
font-family: Arial, Sans-serif;
color: #000000;
font-size: 11px;
text-align: left;
}
...
步骤 7-为 CSS 文件创建基于 Java 的资源文件
package com.tutorialspoint.client;
...
public interface LoginResources extends ClientBundle {
public interface MyCss extends CssResource {
String blackText();
...
}
@Source("Login.css")
MyCss style();
}
第 8 步-在 Java UI 代码文件中附加 CSS 资源。
使用基于 Java 的小部件类的构造函数
Login.java
附加外部 CSS 文件
Login.css
public Login() {
this.res = GWT.create(LoginResources.class);
res.style().ensureInjected();
initWidget(uiBinder.createAndBindUi(this));
}
UIBinder 完整示例
此示例将带您通过简单的步骤来展示 GWT 中 UIBinder 的用法。按照以下步骤更新我们在
GWT-创建应用程序章节中创建的 GWT 应用程序-
步骤 |
描述 |
在com.tutorialspoint 包下创建一个名为HelloWorld 的项目,如GWT-创建应用程序 章节所述。 |
将HelloWorld.gwt.xml、HelloWorld.css、HelloWorld.html和HelloWorld.java修改为下面解释。保持其余文件不变。 |
编译并运行应用程序以验证实现逻辑的结果。 |
以下是修改后的模块描述符
src/com.tutorialspoint/HelloWorld.gwt.xml的内容。
<?xml version = "1.0" encoding = "UTF-8"?>
<module rename-to = 'helloworld'>
<!--Inherit the core Web Toolkit stuff. -->
<inherits name = 'com.google.gwt.user.User'/>
<!--Inherit the default GWT style sheet. -->
<inherits name = 'com.google.gwt.user.theme.clean.Clean'/>
<!--Inherit the UiBinder module. -->
<inherits name = "com.google.gwt.uibinder.UiBinder"/>
<!--Specify the app entry point class. -->
<entry-point class = 'com.tutorialspoint.client.HelloWorld'/>
<!--Specify the paths for translatable code -->
<source path ='client'/>
<source path = 'shared'/>
</module>
以下是修改后的样式表文件
war/HelloWorld.css的内容。
body {
text-align: center;
font-family: verdana, sans-serif;
}
h1 {
font-size: 2em;
font-weight: bold;
color: #777777;
margin: 40px 0px 70px;
text-align: center;
}
以下是修改后的HTML主机文件
war/HelloWorld.html的内容。
<html>
<head>
<title>Hello World</title>
<link rel = "stylesheet" href = "HelloWorld.css"/>
<script language = "javascript" src = "helloworld/helloworld.nocache.js">
</script>
</head>
<body>
<h1>UiBinder Demonstration</h1>
<div id = "gwtContainer"></div>
</body>
</html>
现在创建一个新的 UiBinder 模板和所有者类(文件 → 新建 → UiBinder)。
为项目选择客户端包,然后将其命名为 Login。保留所有其他默认值。单击完成按钮,插件将创建一个新的 UiBinder 模板和所有者类。
现在在
src/com.tutorialspoint/client 包中创建 Login.css 文件并将以下内容放入其中
.blackText {
font-family: Arial, Sans-serif;
color: #000000;
font-size: 11px;
text-align: left;
}
.redText {
font-family: Arial, Sans-serif;
color: #ff0000;
font-size: 11px;
text-align: left;
}
.loginButton {
border: 1px solid #3399DD;
color: #FFFFFF;
background: #555555;
font-size: 11px;
font-weight: bold;
margin: 0 5px 0 0;
padding: 4px 10px 5px;
text-shadow: 0-1px 0 #3399DD;
}
.box {
border: 1px solid #AACCEE;
display: block;
font-size: 12px;
margin: 0 0 5px;
padding: 3px;
width: 203px;
}
.background {
background-color: #999999;
border: 1px none transparent;
color: #000000;
font-size: 11px;
margin-left:-8px;
margin-top: 5px;
padding: 6px;
}
现在在
src/com.tutorialspoint/client 包中创建 LoginResources.java 文件并将以下内容放入其中
package com.tutorialspoint.client;
import com.google.gwt.resources.client.ClientBundle;
import com.google.gwt.resources.client.CssResource;
public interface LoginResources extends ClientBundle {
/**
* Sample CssResource.
*/
public interface MyCss extends CssResource {
String blackText();
String redText();
String loginButton();
String box();
String background();
}
@Source("Login.css")
MyCss style();
}
将
src/com.tutorialspoint/client包中的Login.ui.xml内容替换为以下内容
<ui:UiBinder xmlns:ui = 'urn:ui:com.google.gwt.uibinder'
xmlns:gwt = 'urn:import:com.google.gwt.user.client.ui'
xmlns:res = 'urn:with:com.tutorialspoint.client.LoginResources'>
<ui:with type = "com.tutorialspoint.client.LoginResources" field = "res">
</ui:with>
<gwt:HTMLPanel>
<div align = "center">
<gwt:VerticalPanel res:styleName = "style.background">
<gwt:Label text = "Login" res:styleName = "style.blackText" />
<gwt:TextBox ui:field="loginBox" res:styleName = "style.box" />
<gwt:Label text = "Password" res:styleName = "style.blackText" />
<gwt:PasswordTextBox ui:field = "passwordBox" res:styleName = "style.box" />
<gwt:HorizontalPanel verticalAlignment = "middle">
<gwt:Button ui:field = "buttonSubmit" text="Submit"
res:styleName = "style.loginButton" />
<gwt:CheckBox ui:field = "myCheckBox" />
<gwt:Label ui:field = "myLabel" text = "Remember me"
res:styleName = "style.blackText" />
</gwt:HorizontalPanel>
<gwt:Label ui:field = "completionLabel1" res:styleName = "style.blackText" />
<gwt:Label ui:field = "completionLabel2" res:styleName = "style.blackText" />
</gwt:VerticalPanel>
</div>
</gwt:HTMLPanel>
</ui:UiBinder>
将
src/com.tutorialspoint/client包中Login.java的内容替换为以下内容
package com.tutorialspoint.client;
import com.google.gwt.core.client.GWT;
import com.google.gwt.event.dom.client.ClickEvent;
import com.google.gwt.event.logical.shared.ValueChangeEvent;
import com.google.gwt.uibinder.client.UiBinder;
import com.google.gwt.uibinder.client.UiField;
import com.google.gwt.uibinder.client.UiHandler;
import com.google.gwt.uibinder.client.UiTemplate;
import com.google.gwt.user.client.Window;
import com.google.gwt.user.client.ui.Composite;
import com.google.gwt.user.client.ui.Label;
import com.google.gwt.user.client.ui.TextBox;
import com.google.gwt.user.client.ui.Widget;
public class Login extends Composite {
private static LoginUiBinder uiBinder = GWT.create(LoginUiBinder.class);
/*
* @UiTemplate is not mandatory but allows multiple XML templates
* to be used for the same widget.
* default file loaded will be <class-name>.ui.xml
*/
@UiTemplate("Login.ui.xml")
interface LoginUiBinder extends UiBinder<Widget, Login> {
}
@UiField(provided = true)
final LoginResources res;
public Login() {
this.res = GWT.create(LoginResources.class);
res.style().ensureInjected();
initWidget(uiBinder.createAndBindUi(this));
}
@UiField
TextBox loginBox;
@UiField
TextBox passwordBox;
@UiField
Label completionLabel1;
@UiField
Label completionLabel2;
private boolean tooshort = false;
/*
* Method name is not relevant, the binding is done according to the class
* of the parameter.
*/
@UiHandler("buttonSubmit")
void doClickSubmit(ClickEvent event) {
if (!tooShort) {
Window.alert("Login Successful!");
} else {
Window.alert("Login or Password is too short!");
}
}
@UiHandler("loginBox")
void handleLoginChange(ValueChangeEvent<String> event) {
if (event.getValue().length() < 6) {
completionLabel1.setText("Login too short (Size must be > 6)");
tooshort = true;
} else {
tooshort = false;
completionLabel1.setText("");
}
}
@UiHandler("passwordBox")
void handlePasswordChange(ValueChangeEvent<String> event) {
if (event.getValue().length() < 6) {
tooshort = true;
completionLabel2.setText("Password too short (Size must be > 6)");
} else {
tooshort = false;
completionLabel2.setText("");
}
}
}
让我们看看以下 Java 文件
src/com.tutorialspoint/HelloWorld.java 的内容,它将演示 UiBinder 的使用。
package com.tutorialspoint.client;
import com.google.gwt.core.client.EntryPoint;
import com.google.gwt.user.client.ui.RootPanel;
public class HelloWorld implements EntryPoint {
public void onModuleLoad() {
RootPanel.get().add(new Login());
}
}
一旦您准备好完成所有更改,让我们像在GWT-创建应用程序一章中所做的那样,在开发模式下编译和运行应用程序。如果您的应用程序一切正常,这将产生以下结果-
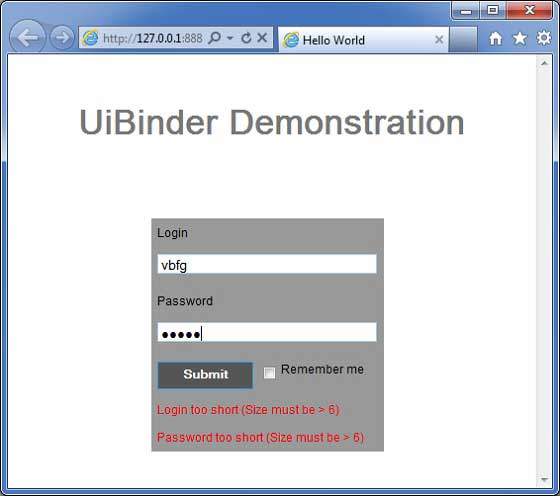